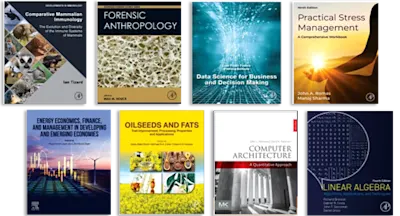
Unit Testing in Java
How Tests Drive the Code
- 1st Edition - May 20, 2003
- Imprint: Morgan Kaufmann
- Author: Johannes Link
- Language: English
- Paperback ISBN:9 7 8 - 1 - 5 5 8 6 0 - 8 6 8 - 9
- eBook ISBN:9 7 8 - 0 - 0 8 - 0 5 2 0 1 7 - 9
Software testing is indispensable and is one of the most discussed topics in software development today. Many companies address this issue by assigning a dedicated software testing… Read more
Purchase options
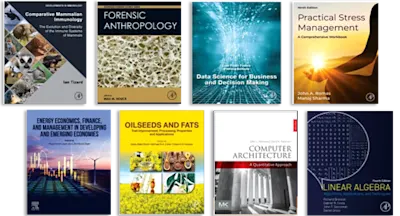
Software testing is indispensable and is one of the most discussed topics in software development today. Many companies address this issue by assigning a dedicated software testing phase towards the end of their development cycle. However, quality cannot be tested into a buggy application. Early and continuous unit testing has been shown to be crucial for high quality software and low defect rates. Yet current books on testing ignore the developer's point of view and give little guidance on how to bring the overwhelming amount of testing theory into practice. Unit Testing in Java represents a practical introduction to unit testing for software developers. It introduces the basic test-first approach and then discusses a large number of special issues and problem cases. The book instructs developers through each step and motivates them to explore further.
- Shows how the discovery and avoidance of software errors is a demanding and creative activity in its own right and can build confidence early in a project.
- Demonstrates how automated tests can detect the unwanted effects of small changes in code within the entire system.
- Discusses how testing works with persistency, concurrency, distribution, and web applications.
- Includes a discussion of testing with C++ and Smalltalk.
Software developers and Java programmers.
Part 1: Basic Techniques1 Introduction1.1 Important Terms1.2 XP Testing1.3 Classic Testing1.4 "Test-First Development" - A Brief Definition1.5 Java Only - Or Other Coffee?1.6 Objectives of This Book1.7 Organization of This Book1.8 Conventions in This Book1.9 Web Site to This Book2 Automating Unit Tests2.1 What Do We Want to Automate?2.2 Requirements to an Automation Framework2.3 Junit2.4 Summary3 Basic Steps of the Test-first Approach3.1 Step by Step3.2 Dependencies3.3 Organizing and Running Tests3.4 Summary4 Test Ideas and Heuristics4.1 Reworking Single Tests4.2 Black and White Boxes4.3 Testing the Typical Functionality4.4 Threshold Values and Equivalence Classes4.5 Error Cases and Exceptions4.6 Object Interactions4.7 Design by Contract4.8 More Ideas to Find Test Cases4.9 Refactoring Code and Tests4.10 Summary5 The Inner Life of a Test Framework5.1 Statics5.2 The Life Cycle of a Test Suite5.3 Project-specific Expansions5.4 Summary6 Dummy and Mock Objects for Independence6.1 Little Dummies6.2 Weltering in Technical Terms6.3 Big Dummies6.4 Extending our Mansion6.5 Endoscopic Testing6.6 Mock Objects from the Assembly Line6.7 Testing Threshold Values and Exceptions6.8 How Does the Test Get to the Mock?6.9 Evil Singletons6.10 Lightweight and Heavyweight Mocks6.11 File Dummies6.12 More Typical Mock Objects6.13 External Components6.14 The Pros and Cons6.15 Summary7 Inheritance and Polymorphism7.1 Inheritance7.2 Polymorphism7.3 Summary8. How Much is Enough?8.1 The XP Rule8.2 Clear Answers to Clear Questions8.3 Test Coverage8.4 SummaryPart II: Advanced Topics9 Persistent Objects9.1 Abstract Persistence Interface9.2 Persistent Dummy9.3 Designing a Database Interface9.4 Testing the "Right" Persistence9.5 Interaction Between Persistence Layer and Client9.6 Summary10 Concurrent Programs10.1 Problems Using Threads10.2 Testing Asynchronous Services10.3 Testing for Synchronization10.4 Summary11 Distributed Applications11.1 RMI11.2 Enterprise JavaBeans11.3 Summary12 Web Applications12.1 Functional Tests12.2 Testing on the Server12.3 Testing with Dummies12.4 Separating the Servlet API from the Servlet Logic12.5 Testing the HTML Generation12.6 Summary13 Graphical User Interfaces13.1 The Direct Way13.2 Short Detours13.3 Summary14 The Role of Unit Tests in the Software Process14.1 Activities in the Defined Software Process14.2 Process Types and Testing Strategies14.3 Costs and Benefits of Automated Unit Tests14.4 Commercial Process Models14.5 Will Automated Unit Tests Fit in My Process?15 Loose Ends and Opportunities15.1 Unit Testing for Existing Software15.2 Introducing Unit Tests to the Development Team15.3 What's MissingPart III: AppendixA. Notes to JUnitB. Unit Tests with Other Programming LanguagesC. GlossaryD. Bibliography & References
- Edition: 1
- Published: May 20, 2003
- Imprint: Morgan Kaufmann
- Language: English
JL
Johannes Link
For 4 years Johannes Link has been project manager and software developer at andrena objects ag in Karlsruhe, Germany. He came to andrena after years of practical software engineering research at the German Cancer Research Center and the German ABB Corporate Research Center. Johannes is responsible for andrena's internal and external training activities and has published articles on software testing and software development. He holds a diploma degree in medical computer science from Heidelberg University.
Affiliations and expertise
andrena objects ag, Karlsruhe, Germany.Read Unit Testing in Java on ScienceDirect