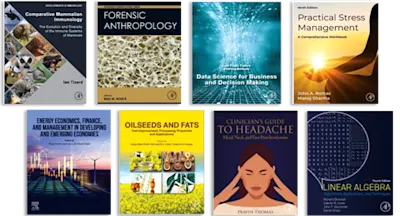
Understanding UML
The Developer's Guide
- 1st Edition - October 1, 1997
- Imprint: Morgan Kaufmann
- Author: Mark Watson
- Language: English
- Paperback ISBN:9 7 8 - 1 - 5 5 8 6 0 - 4 6 5 - 0
- eBook ISBN:9 7 8 - 0 - 0 8 - 0 5 2 0 0 7 - 0
The Unified Modeling Language (UML) is a third generation method for specifying, visualizing, and documenting an object-oriented system under development. It unifies the three… Read more
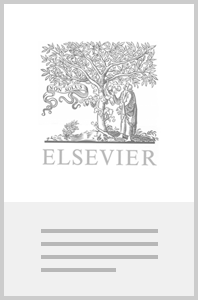
Purchase options
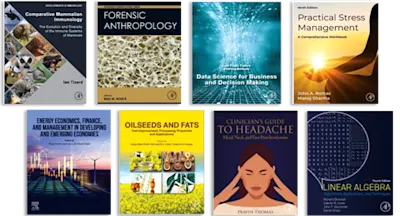
Institutional subscription on ScienceDirect
Request a sales quoteThe Unified Modeling Language (UML) is a third generation method for specifying, visualizing, and documenting an object-oriented system under development. It unifies the three leading object-oriented methods and others to serve as the basis for a common, stable, and expressive object-oriented development notation. As the complexity of software applications increases, so does the developer's need to design and analyze applications before developing them. This practical introduction to UML provides software developers with an overview of this powerful new design notation, and teaches Java programmers to analyse and design object-oriented applications using the UML notation.
- Apply the basics of UML to your applications immediately, without having to wade through voluminous documentation
- Use the simple Internet example as a prototype for developing object-oriented applications of your own
- Follow a real example of an Intranet sales reporting system written in Java that is used to drive explanations throughout the book
- Learn from an example application modeled both by hand and with the use of Popkin Software's SA/Object Architect O-O visual modeling tool.
1 Introduction
1.1 Development of UML
1.2 Using UML to Develop Applications
1.3 How This Book is Organized
2 The Vocabulary of Object Technology
2.1 What Are Objects?
2.2 Objects, Classes, and Instances
2.3 Message Passing and Associations
2.4 Class Hierarchies and Class Inheritance
2.5 Public, Private, and Protected
2.6 Interface Inheritance
2.7 Polymorphism
2.8 Beyond the Basics
3 A Simple Object-Oriented Methodology
3.1 An Iterative Approach to Object-Oriented Development
3.2 The Phases of a Development Cycle
3.2.1 Requirements Analysis
3.2.2 The Analysis Phase
3.2.3 The Design Phase
3.2.4 The Coding Phase
3.2.5 The Testing Phase
4 The Unified Modeling Language
4.1 UML Diagrams
4.1.1 Use Case Diagrams
4.1.2 Static Structure Diagrams
4.1.3 Interaction Diagrams
4.1.4 State Diagrams
4.1.5 Implementation Diagrams
4.1.6 The Diagramming Process
4.2 Additional Diagrams and Notations
4.3 A Generic Approach to Object-Oriented Development
4.4 A UML Notation Job Aid
5 Coding Applications in Java
5.1 Java As an Object-Oriented Language
5.1.1 Java Language Basics
5.2 The Java Development Kit
5.3 The Java Platform
5.4 Compiling and Interpreting Java
5.4.1 Applets versus Applications
5.4.2 JavaBeans
6 Java Development with an Object-Oriented Modeling Tool
6.1 Object-Oriented Modeling Tools
6.2 Popkin Software's SA/Object Architect
6.2.2 Putting It All Together
7 Business Process Reengineering
7.1 What is Business Process Reengineering
7.1.2 The Redesign Process
7.2 IBM's Line of Vision Enterprise Methodology Diagrams and Notation
7.3 Watson's Existing Sales Process
7.4 Watson's Reengineered Sales Process
7.5 Automating the BPR Process
8 Use Case Diagrams and Ideal Object Models
8.1 Use Case Diagrams
8.2 Use Cases and Business Process Reengineering
8.3 A Use Case Diagram of the Watson's SalesWeb System
8.3.1 Clarifying Our Vocabulary Regarding the Watson's Application
8.4 Use Case Descriptions
8.5 Instances of Use Cases As Test Cases
8.6 From Use Cases to Ideal Object Models
8.7 The OOSE Ideal Object Model
8.7.1 Interface Classes
8.7.2 Entity Classes
8.7.3 Control Classes
8.7.4 Identifying Classes in Use Cases
8.8 An Ideal Object Model for the Report Sales Use Case
8.9 Creating Use Case Models with an Object-Oriented Modeling Tool
9 CRC Cards
9.1 The Layout of a CRC Card
9.2 The Steps in a CRC Session
9.2.1 Step 1: Assemble a Group
9.2.2 Step 2: Review Requirements
9.2.3 Step 3: Brainstorm a List of Classes
9.2.4 Step 4: Review the List of Classes
9.2.5 Step 5: Prepare CRC Cards
9.2.6 Step 6: Develop a Description of Each Class
9.2.7 Step 7: Brainstorm Responsibilities and Collaborators
9.2.8 Step 8: Generate Specific Scenarios
9.2.9 Step 9: Talk Through Several Scenarios
9.3 CRC Cards and Object-Oriented Thinking
9.4 Terms versus UML Terms
9.5 Automating the CRC Process
10 UML Class and Object Diagrams
10.1 Class Diagramming Basics
10.1.1 Attributes (Variables)
10.1.2 Operations (Methods)
10.1.3 More on Attributes and Operations
10.1.4 Associations
10.1.5 Class Inheritance
10.1.6 Interfaces
10.1.7 Identifying Aggregations
10.1.8 Constraints and Notes
10.2 Diagramming Objects
10.3 Creating a Class Diagram
10.3.1 An Object Diagram
10.3.2 A More Elaborate Class Diagram
10.4 Creating UML Class Diagrams with and Object-Oriented Modeling Tool
11 UML Sequence and Collaboration Diagrams
11.2 Sequence Diagrams
11.2.1 Sequence Notation
11.2.2 Creating a Sequence Diagram
11.3 Collaboration Diagrams
11.3.1 Collaboration Notation
11.3.2 Creating a Collaboration Diagram
11.3.3 Collaboration Diagrams and Patterns
11.4 Creating Sequence and Collaboration Diagrams with an Object-Oriented Modeling Tool
12 UML State and Activity Diagrams
12.1 State Diagrams
12.1.1 State Diagram Notation
12.1.2 Creating a State Diagram
12.2 Activity Diagrams
12.3 Creating State and Activity Diagrams with an Object-Oriented Modeling CASE Tool
13 Designing an Object-Oriented System
13.1 Moving from Analysis to Design
14 Choosing an Object-Oriented Architecture
14.1 Dividing an Application into Tiers
14.1.1 A Two-Tiered Design
14.1.2 A Three-Tiered Design
14.2 Assigning Packages to Tiers and Platforms
14.2.1 Two- and Three-Tiered Class Diagrams
14.3 UML Implementation Diagrams
14.4 Linking the Tiers
14.4.1 Object Request Brokers
14.5 Summing Up
14.6 The Architecture of the SalesWeb System
14.7 Capturing a Design in an Object-Oriented Modeling Tool
15 Expanding Your Design
15.1 Expanding Your Object Model
15.1.1 Extending Your Analysis Diagrams
15.1.2 Getting Concrete About Operations
15.1.3 Patterns
15.1.4 Using Classes, Interfaces, and Components
15.2 Developing User Screens or Web Pages
15.3 Arranging to Access Data
15.3.1 The Java DataBase Connectivity Package
15.3.2 Object-Oriented and Object-Relational Databases
15.4 The SalesWeb Design
15.5 Coding and Testing
15.5.1 Bottom-Up Testing Starts with Classes
15.5.2 Top-Down Testing Starts with Use Cases
15.6 Expanding Your Design in an Object-Oriented Modeling Tool
Appendix A Code for the SalesWeb Example
Appendix B A Comparison of UML, Booch, and MOT Notations
Appendix C Products Mentioned in the Book
Bibliography, Notes, and Web Sites
Index
1.1 Development of UML
1.2 Using UML to Develop Applications
1.3 How This Book is Organized
2 The Vocabulary of Object Technology
2.1 What Are Objects?
2.2 Objects, Classes, and Instances
2.3 Message Passing and Associations
2.4 Class Hierarchies and Class Inheritance
2.5 Public, Private, and Protected
2.6 Interface Inheritance
2.7 Polymorphism
2.8 Beyond the Basics
3 A Simple Object-Oriented Methodology
3.1 An Iterative Approach to Object-Oriented Development
3.2 The Phases of a Development Cycle
3.2.1 Requirements Analysis
3.2.2 The Analysis Phase
3.2.3 The Design Phase
3.2.4 The Coding Phase
3.2.5 The Testing Phase
4 The Unified Modeling Language
4.1 UML Diagrams
4.1.1 Use Case Diagrams
4.1.2 Static Structure Diagrams
4.1.3 Interaction Diagrams
4.1.4 State Diagrams
4.1.5 Implementation Diagrams
4.1.6 The Diagramming Process
4.2 Additional Diagrams and Notations
4.3 A Generic Approach to Object-Oriented Development
4.4 A UML Notation Job Aid
5 Coding Applications in Java
5.1 Java As an Object-Oriented Language
5.1.1 Java Language Basics
5.2 The Java Development Kit
5.3 The Java Platform
5.4 Compiling and Interpreting Java
5.4.1 Applets versus Applications
5.4.2 JavaBeans
6 Java Development with an Object-Oriented Modeling Tool
6.1 Object-Oriented Modeling Tools
6.2 Popkin Software's SA/Object Architect
6.2.2 Putting It All Together
7 Business Process Reengineering
7.1 What is Business Process Reengineering
7.1.2 The Redesign Process
7.2 IBM's Line of Vision Enterprise Methodology Diagrams and Notation
7.3 Watson's Existing Sales Process
7.4 Watson's Reengineered Sales Process
7.5 Automating the BPR Process
8 Use Case Diagrams and Ideal Object Models
8.1 Use Case Diagrams
8.2 Use Cases and Business Process Reengineering
8.3 A Use Case Diagram of the Watson's SalesWeb System
8.3.1 Clarifying Our Vocabulary Regarding the Watson's Application
8.4 Use Case Descriptions
8.5 Instances of Use Cases As Test Cases
8.6 From Use Cases to Ideal Object Models
8.7 The OOSE Ideal Object Model
8.7.1 Interface Classes
8.7.2 Entity Classes
8.7.3 Control Classes
8.7.4 Identifying Classes in Use Cases
8.8 An Ideal Object Model for the Report Sales Use Case
8.9 Creating Use Case Models with an Object-Oriented Modeling Tool
9 CRC Cards
9.1 The Layout of a CRC Card
9.2 The Steps in a CRC Session
9.2.1 Step 1: Assemble a Group
9.2.2 Step 2: Review Requirements
9.2.3 Step 3: Brainstorm a List of Classes
9.2.4 Step 4: Review the List of Classes
9.2.5 Step 5: Prepare CRC Cards
9.2.6 Step 6: Develop a Description of Each Class
9.2.7 Step 7: Brainstorm Responsibilities and Collaborators
9.2.8 Step 8: Generate Specific Scenarios
9.2.9 Step 9: Talk Through Several Scenarios
9.3 CRC Cards and Object-Oriented Thinking
9.4 Terms versus UML Terms
9.5 Automating the CRC Process
10 UML Class and Object Diagrams
10.1 Class Diagramming Basics
10.1.1 Attributes (Variables)
10.1.2 Operations (Methods)
10.1.3 More on Attributes and Operations
10.1.4 Associations
10.1.5 Class Inheritance
10.1.6 Interfaces
10.1.7 Identifying Aggregations
10.1.8 Constraints and Notes
10.2 Diagramming Objects
10.3 Creating a Class Diagram
10.3.1 An Object Diagram
10.3.2 A More Elaborate Class Diagram
10.4 Creating UML Class Diagrams with and Object-Oriented Modeling Tool
11 UML Sequence and Collaboration Diagrams
11.2 Sequence Diagrams
11.2.1 Sequence Notation
11.2.2 Creating a Sequence Diagram
11.3 Collaboration Diagrams
11.3.1 Collaboration Notation
11.3.2 Creating a Collaboration Diagram
11.3.3 Collaboration Diagrams and Patterns
11.4 Creating Sequence and Collaboration Diagrams with an Object-Oriented Modeling Tool
12 UML State and Activity Diagrams
12.1 State Diagrams
12.1.1 State Diagram Notation
12.1.2 Creating a State Diagram
12.2 Activity Diagrams
12.3 Creating State and Activity Diagrams with an Object-Oriented Modeling CASE Tool
13 Designing an Object-Oriented System
13.1 Moving from Analysis to Design
14 Choosing an Object-Oriented Architecture
14.1 Dividing an Application into Tiers
14.1.1 A Two-Tiered Design
14.1.2 A Three-Tiered Design
14.2 Assigning Packages to Tiers and Platforms
14.2.1 Two- and Three-Tiered Class Diagrams
14.3 UML Implementation Diagrams
14.4 Linking the Tiers
14.4.1 Object Request Brokers
14.5 Summing Up
14.6 The Architecture of the SalesWeb System
14.7 Capturing a Design in an Object-Oriented Modeling Tool
15 Expanding Your Design
15.1 Expanding Your Object Model
15.1.1 Extending Your Analysis Diagrams
15.1.2 Getting Concrete About Operations
15.1.3 Patterns
15.1.4 Using Classes, Interfaces, and Components
15.2 Developing User Screens or Web Pages
15.3 Arranging to Access Data
15.3.1 The Java DataBase Connectivity Package
15.3.2 Object-Oriented and Object-Relational Databases
15.4 The SalesWeb Design
15.5 Coding and Testing
15.5.1 Bottom-Up Testing Starts with Classes
15.5.2 Top-Down Testing Starts with Use Cases
15.6 Expanding Your Design in an Object-Oriented Modeling Tool
Appendix A Code for the SalesWeb Example
Appendix B A Comparison of UML, Booch, and MOT Notations
Appendix C Products Mentioned in the Book
Bibliography, Notes, and Web Sites
Index
- Edition: 1
- Published: October 1, 1997
- Imprint: Morgan Kaufmann
- Language: English
- Paperback ISBN: 9781558604650
- eBook ISBN: 9780080520070
MW
Mark Watson
Mark Watson is an independent software developer with extensive software engineering experience. He has worked at Angel Studios as a game programmer for Nintendo and Windows 95 games, with SAIC on the development of tools for expert systems, and on natural language processing and neural network systems. He is the developer of a real-time distributed expert system used by regional telephone systems to detect fraud, and is the author of eight books.