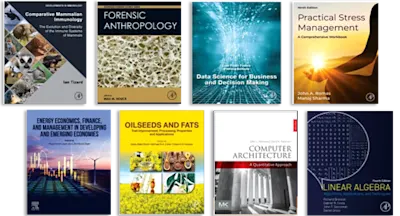
Programming Massively Parallel Processors
A Hands-on Approach
- 4th Edition - August 4, 2022
- Imprint: Morgan Kaufmann
- Authors: Wen-mei W. Hwu, David B. Kirk, Izzat El Hajj
- Language: English
- Paperback ISBN:9 7 8 - 0 - 3 2 3 - 9 1 2 3 1 - 0
- eBook ISBN:9 7 8 - 0 - 3 2 3 - 9 8 4 6 3 - 8
Programming Massively Parallel Processors: A Hands-on Approach shows both students and professionals alike the basic concepts of parallel programming and GPU architect… Read more
Purchase options
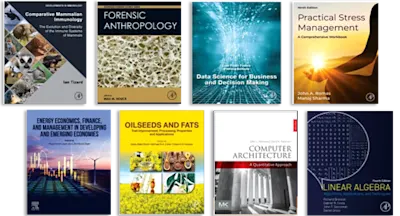
Programming Massively Parallel Processors: A Hands-on Approach shows both students and professionals alike the basic concepts of parallel programming and GPU architecture. Concise, intuitive, and practical, it is based on years of road-testing in the authors' own parallel computing courses. Various techniques for constructing and optimizing parallel programs are explored in detail, while case studies demonstrate the development process, which begins with computational thinking and ends with effective and efficient parallel programs. The new edition includes updated coverage of CUDA, including the newer libraries such as CuDNN. New chapters on frequently used parallel patterns have been added, and case studies have been updated to reflect current industry practices.
- Parallel Patterns Introduces new chapters on frequently used parallel patterns (stencil, reduction, sorting) and major improvements to previous chapters (convolution, histogram, sparse matrices, graph traversal, deep learning)
- Ampere Includes a new chapter focused on GPU architecture and draws examples from recent architecture generations, including Ampere
- Systematic Approach Incorporates major improvements to abstract discussions of problem decomposition strategies and performance considerations, with a new optimization checklist
1 Introduction
Part I Fundamental Concepts
2 Heterogeneous data parallel computing
3 Multidimensional grids and data
4 Compute architecture and scheduling
5 Memory architecture and data locality
6 Performance considerations
Part II Parallel Patterns
7 Convolution: An introduction to constant memory and caching
8 Stencil
9 Parallel histogram
10 Reduction And minimizing divergence
11 Prefix sum (scan)
12 Merge: An introduction to dynamic input data identification
Part III Advanced patterns and applications
13 Sorting
14 Sparse matrix computation
15 Graph traversal
16 Deep learning
17 Iterative magnetic resonance imaging reconstruction
18 Electrostatic potential map
19 Parallel programming and computational thinking
Part IV Advanced Practices
20 Programming a heterogeneous computing cluster: An introduction to CUDA streams
21 CUDA dynamic parallelism
22 Advanced practices and future evolution
23 Conclusion and outlook
Appendix A: Numerical considerations
- Edition: 4
- Published: August 4, 2022
- Imprint: Morgan Kaufmann
- Language: English
WH
Wen-mei W. Hwu
DK
David B. Kirk
At NVIDIA, Kirk led graphics-technology development for some of today's most popular consumer-entertainment platforms, playing a key role in providing mass-market graphics capabilities previously available only on workstations costing hundreds of thousands of dollars. For his role in bringing high-performance graphics to personal computers, Kirk received the 2002 Computer Graphics Achievement Award from the Association for Computing Machinery and the Special Interest Group on Graphics and Interactive Technology (ACM SIGGRAPH) and, in 2006, was elected to the National Academy of Engineering, one of the highest professional distinctions for engineers.
Kirk holds 50 patents and patent applications relating to graphics design and has published more than 50 articles on graphics technology, won several best-paper awards, and edited the book Graphics Gems III. A technological "evangelist" who cares deeply about education, he has supported new curriculum initiatives at Caltech and has been a frequent university lecturer and conference keynote speaker worldwide.
IE