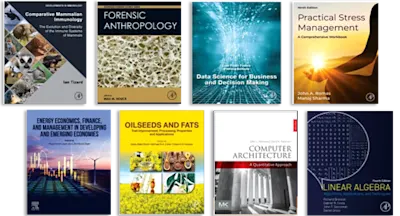
Pocket Guide to TCP/IP Socket Programming in C
- 1st Edition - September 6, 2000
- Imprint: Morgan Kaufmann
- Authors: Michael J. Donahoo, Kenneth L. Calvert
- Language: English
- Paperback ISBN:9 7 8 - 1 - 5 5 8 6 0 - 6 8 6 - 9
- eBook ISBN:9 7 8 - 0 - 0 8 - 0 5 7 4 1 2 - 7
The Pocket Guide to TCP/IP Sockets is a quick and affordable way to gain the knowledge and skills you need to develop sophisticated and powerful networked-based programs usin… Read more
Purchase options
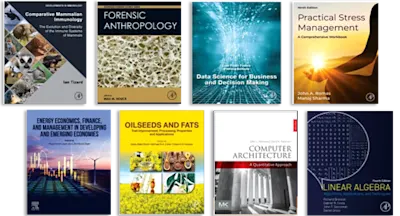
The Pocket Guide to TCP/IP Sockets is a quick and affordable way to gain the knowledge and skills you need to develop sophisticated and powerful networked-based programs using sockets. Written by two experienced networking instructors, this book provides a series of examples that demonstrate basic sockets techniques for clients and servers.
Using plenty of real-world examples, this book is a complete beginner's guide to socket programming and a springboard to more advanced networking topics, including multimedia protocols.
* Comprehensive example-based coverage of the most important TCP/IP techniques-including iterative and concurrent servers, timeouts, and asynchronous message processing.
* Includes a detailed, easy-to-use reference to the system calls and auxiliary routines that comprise the sockets interface.
* A companion Web site provides source code for all example programs in both C and WinSock versions, as well as guidance on running the code on various platforms.
I Tutorial
1 Introduction
1.1 Networks, Packets and Protocols
1.2 About Addresses
1.3 Clients and Servers
1.4 What is a Socket?
2 Basic Sockets
2.1 Creating and Destroying
2.2 Specifying Addresses
2.3 TCP Client
2.4 TCP Server
3 Constructing Messages
3.1 Encoding Data
3.2 Byte Ordering
3.3 Alignment and Padding
3.4 Framing and Parsing
4 Using UDP Sockets
4.1 UDP Client
4.2 UDP Server
4.3 Sending and Receiving with UDP Sockets
5 Socket Programming
5.1 Socket Options
5.2 Signals
5.3 Nonblocking I/O
5.3.1 Nonblocking Sockets
5.3.2 Asynchronous I/O
5.3.3 Timeouts
5.4 Multitasking
5.4.1 Per-client Processes
5.4.2 Per-client Thread
5.4.3 Constrained-Multitasking
5.5 Multiplexing
5.6 Multiple Recipients
5.6.1 Broadcast
5.6.2 Multicast
5.6.3 Broadcast vs. Multicast
6 Under The Hood
6.1 Buffering and TCP
6.2 Deadlock
6.3 Performance Implications
6.4 TCP Socket Life Cycle
6.4.1 Connecting
6.4.2 Closing A TCP Connection
6.5 Demultiplexing Demystified
7 Domain Name Service
7.1 Mapping Between Names and Internet addresses
7.2 Finding Service Information by Name
II API Reference
Data Structures
sockaddr
sockaddr_in
Socket Setup
socket()
bind()
getsockname()
Socket Connection
connect()
listen()
accept()
getpeername()
Socket Communication
send()
sendto()
recv()
recvfrom()
close()
shutdown()
Socket Control
getsockopt()
setsockopt()
Binary/String Conversion
inet_ntoa()
inet_addr()
htons(), htonl(), ntohs(), ntohl()
Host and Service Information
gethostname()
gethostbyname()
gethostbyaddr()
getservbyname()
getservbyport()
- Edition: 1
- Published: September 6, 2000
- Imprint: Morgan Kaufmann
- Language: English
MD
Michael J. Donahoo
Michael J. Donahoo teaches networking to undergraduate and graduate students at Baylor University, where he is an assistant professor. He received his Ph.D. in computer science from the Georgia Institute of Technology. His research interests are in large-scale information dissemination and management.
KC
Kenneth L. Calvert
Kenneth L. Calvert is an associate professor at University of Kentucky, where he teaches and does research on the design and implementation of computer network protocols. He has been doing networking research since 1987, and teaching since 1991. He holds degrees from MIT, Stanford, and the University of Texas at Austin.