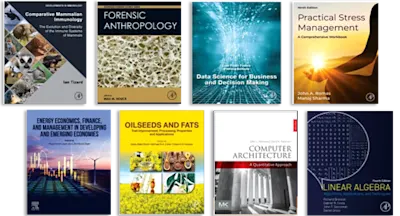
Multicore and GPU Programming
An Integrated Approach
- 2nd Edition - February 9, 2022
- Imprint: Morgan Kaufmann
- Author: Gerassimos Barlas
- Language: English
- Paperback ISBN:9 7 8 - 0 - 1 2 - 8 1 4 1 2 0 - 5
- eBook ISBN:9 7 8 - 0 - 1 2 - 8 1 4 1 2 1 - 2
Multicore and GPU Programming: An Integrated Approach, Second Edition offers broad coverage of key parallel computing tools, essential for multi-core CPU programming and many-core… Read more
Purchase options
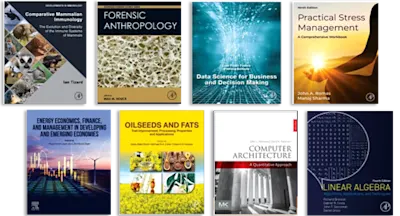
Multicore and GPU Programming: An Integrated Approach, Second Edition offers broad coverage of key parallel computing tools, essential for multi-core CPU programming and many-core "massively parallel" computing. Using threads, OpenMP, MPI, CUDA and other state-of-the-art tools, the book teaches the design and development of software capable of taking advantage of modern computing platforms that incorporate CPUs, GPUs and other accelerators.
Presenting material refined over more than two decades of teaching parallel computing, author Gerassimos Barlas minimizes the challenge of transitioning from sequential programming to mastering parallel platforms with multiple examples, extensive case studies, and full source code. By using this book, readers will better understand how to develop programs that run over distributed memory machines using MPI, create multi-threaded applications with either libraries or directives, write optimized applications that balance the workload between available computing resources, and profile and debug programs targeting parallel machines.
- Includes comprehensive coverage of all major multi-core and many-core programming tools and platforms, including threads, OpenMP, MPI, CUDA, OpenCL and Thrust
- Covers the most recent versions of the above at the time of publication
- Demonstrates parallel programming design patterns and examples of how different tools and paradigms can be integrated for superior performance
- Updates in the second edition include the use of the C++17 standard for all sample code, a new chapter on concurrent data structures, a new chapter on OpenCL, and the latest research on load balancing
- Includes downloadable source code, examples and instructor support materials on the book’s companion website
Part A: Introduction
1. Introduction
2. Multicore and Parallel Program Design
Part B: Programming with Threads and Processes
3. Shared-memory Programming: Threads
4. Concurrent Data Structures
5. Distributed Memory Programming MPI
6. GPU Programming: CUDA
7. GPU Programming: OpenCL
Part C: Higher-level Programming
8. Shared-memory Programming: OpenMP
9. GPU Programming: OpenACC
10. The Thrust Template Library
Part D: Advanced Topics
11. Load Balancing
- Edition: 2
- Published: February 9, 2022
- Imprint: Morgan Kaufmann
- Language: English
GB