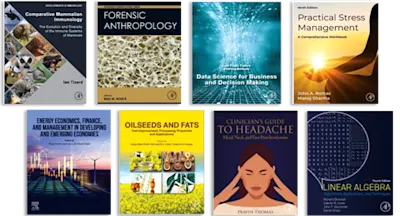
Efficient C/C++ Programming
Smaller, Faster, Better
- 2nd Edition - October 21, 1994
- Imprint: Academic Press
- Author: Steve Heller
- Language: English
- Paperback ISBN:9 7 8 - 0 - 1 2 - 3 3 9 0 9 5 - 0
- eBook ISBN:9 7 8 - 1 - 4 8 3 2 - 6 5 6 3 - 6
Efficient C/C++ Programming describes a practical, real-world approach to efficient C/C++ programming. Topics covered range from how to save storage using a restricted character… Read more
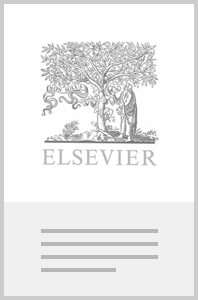
Purchase options
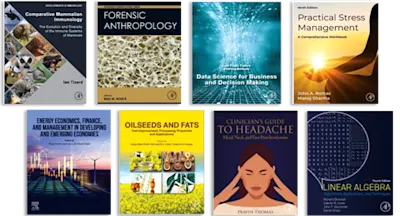
Institutional subscription on ScienceDirect
Request a sales quoteEfficient C/C++ Programming describes a practical, real-world approach to efficient C/C++ programming. Topics covered range from how to save storage using a restricted character set and how to speed up access to records by employing hash coding and caching. A selective mailing list system is used to illustrate rapid access to and rearrangement of information selected by criteria specified at runtime. Comprised of eight chapters, this book begins by discussing factors to consider when deciding whether a program needs optimization. In the next chapter, a supermarket price lookup system is used to illustrate how to save storage by using a restricted character set and how to speed up access to records with the aid of hash coding and caching. Attention is paid to rapid retrieval of prices. A selective mailing list system is then used to illustrate rapid access to and rearrangement of information selected by criteria specified at runtime. The book also considers the Huffman coding and arithmetic coding methods of data compression; a token-threaded interpreter whose code can run faster than equivalent compiled C code, due to its greater code density; a customer database program with variable-length records; and index and key access to variable-length records. The final chapter summarizes the characteristics of the algorithms encountered in previous chapters, as well as the future of the art of optimization. This monograph will be a useful resource for practicing computer programmers and those who intend to be working programmers.
Table of Listings
Foreword
Preface
Acknowledgments
1 Let's Get Small (and Fast): Introduction to Optimization
Deciding Whether to Optimize
Why Optimization is Necessary
Why Optimization is Often Neglected
Considering a Hardware Solution
Categories of Optimization
Finding the Critical Resource
Determining How Much Optimization Is Needed
A Real-Life Example
Summary
2 Hash, Cache and Crunch: A Supermarket Price Lookup System
Introduction
Up the Down Staircase
Some Random Musings
Hashing It Out
Divide and Conquer
Unite and Rule
Knowing When to Stop
Handling Subfile Overflow
Some Drawbacks of Hashing
Caching out Our Winnings
Heading for The Final Lookup
Saving Storage
The Code
Some User-Defined Types
Preparing to Access the Price File
Making a Hash of Things
Searching the File
Wrapping Around at EOF
Summary
Problems
3 Strips, Bits, And Sorts: A Mailing List System
Introduction
A First Approach
Saving Storage with Bitmaps
Increasing Processing Speed
The Law of Diminishing Returns
Strip Mining
Sorting Speed
The Distribution Counting Sort
Multicharacter Sorting
On the Home Stretch
The Code
Determining the Proper Buffer Size
Preparing to Read the Key File
Reading the Key File
Setting a Bit in a Bitmap
Allocate as You Go
Getting Ready to Sort
The Megasort Routine
Finishing Up
Performance
Back to the Future
Moral Support
Summary
Problems
4 Cn U Rd Ths Qkly?: A Data Compression Utility
Introduction
Huffman Coding
Half a Bit Is Better than One
Getting a Bit Excited
A Character Study
Keeping It in Context
Conspicuous Nonconsumption
Receiving the Message
The Code
A Loose Translation
Getting in on the Ground Floor
Gentlemen, Start Your Output
The Main Loop
Finding the Bottlenecks
Dead Slow Ahead
Getting the Correct Answer, Slowly
Some Assembly Is Required
Memories Are Made of This
Loop, De-Loop
Odd Man Out
Getting There Is Half the Fun
A Bunch of Real Characters
1994: A Space Odyssey?
Summary
Problems
5 Do You Need an Interpreter?
Introduction
Lost in Translation
Go FORTH and Multiply
What's in a Name?
The Impossible Dream
Floating Alone
386 Quick Avenue
Caveat Programmer
Timing Is Everything
Summary
Auxiliary Programs
Problems
6 Free at Last: A Customer Database Program with Variable-Length Records
Introduction
A Harmless Fixation
Checking out of the Hotel Procrustes
The Quantum File Access Method
A Large Array
Many Large Arrays
The Light at the End of the Tunnel
The Quantum File Header Record
The Itinerary
Let's Get Physical
A Logical Analysis
The Grab Bag
Taking It from the Top
Summary
Problems
7 OO, What an Algorithm Index and Key Access to Variable-Length Records
Introduction
Rewriting History
Warning: Overload
Hellot Operator?
An Auto-Loader
Type-Safety First
Hidden Virtues
Residence Permit
Punching the Time Clock
Getting Our Clocks Cleaned
Speed Demon
Virtual Perfection
Open Season
The Crown of Creation
Free the Quantum 16K!
One Size Fits All
Well-Founded Assertions
Running on Empty
Assembly Instructions Included
The Top and the Bottom
Where the Wild Goose Goes
Odd Man Out
The Code
Other Assembly Routines
Paying the Piper
Heavenly Hash
Just Another Link in the Chain
Relocation Assistance
Making a Quantum Leap
Persistence Pays Off
In Resplendent Array
Some Fine Details
Bigger and Better
The More, the Merrier
Summary
Problems
8 Mozart, No. Would You Believe Gershwin?
Introduction
Summary of Characteristics
Some Thoughts on the Future
Why Johnny Can't Estimate
Goodbye for Now
Suggested Approaches to Problems
Index
- Edition: 2
- Published: October 21, 1994
- Imprint: Academic Press
- No. of pages: 438
- Language: English
- Paperback ISBN: 9780123390950
- eBook ISBN: 9781483265636
SH
Steve Heller
Steve Heller has been a professional programmer for about 25 years, and is the President of Chrysalis Software Corporation, a consulting firm specializing in high-performance software, and practical, down-to-earth instructional materials. He is the author of two excellent books, Efficient C/C++ Programming and Who’s Afraid of C++?.
Affiliations and expertise
President, Chrysalis Software Corp.Read Efficient C/C++ Programming on ScienceDirect