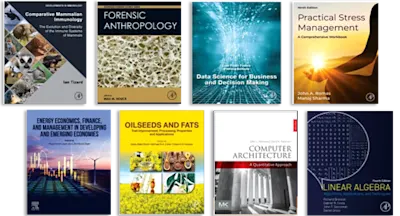
Developing User Interfaces
- 1st Edition - July 1, 1998
- Imprint: Morgan Kaufmann
- Author: Dan R. Olsen
- Language: English
- Paperback ISBN:9 7 8 - 1 - 5 5 8 6 0 - 4 1 8 - 6
- eBook ISBN:9 7 8 - 0 - 0 8 - 0 5 0 4 1 7 - 9
In the early days of computing, technicians in white coats controlled refrigerator-sized computers housed in sealed rooms, far from ordinary users. Today, computers are in… Read more
Purchase options
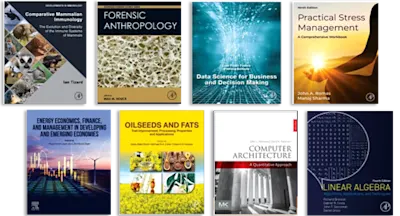
In the early days of computing, technicians in white coats controlled refrigerator-sized computers housed in sealed rooms, far from ordinary users. Today, computers are inexpensive commodities, like television sets,and ordinary people control and interact with them. This new paradigm has led to a burgeoning demand for graphics-intensive and highly interactive interfaces.
Developing User Interfaces is targeted at the programmer who will actually implement, rather than design, the user interface. Most user interface books focus on psychology and usability, not programming techniques. This book recognizes the need for programmers to collaborate with usability experts and psychologists, so topics such as the principles of visualization, human perception, and usability evaluation are touched upon. Yet the primary focus remains on those tools and techniques required for programming the complex user interface.
* Focuses on advanced programming topics* event handling* interaction with geometric objects* widget tool kits* input syntax* Useful to programmers using any language—no particular windowing system or tool kit is presumed, examples are drawn from a variety of commercial systems, and code examples are presented in pseudo code* The basic concepts of traditional computer graphics such as drawing and three-dimensional modeling are covered for readers without a computer graphics background.
- Preface
Chapter 1 Introduction
1.1 What This Book Is About
- 1.1.1 Early Computing
1.1.2 Winds of Change
1.1.3 The Legacy of Lab Coats
1.1.4 A Question of Control
1.2 Setting the Context
- 1.2.1 Computer Graphics
1.2.2 Human Factors and Usability
1.2.3 Object-Oriented Software
1.2.4 Commercial Tools
1.3 An Overview of the User Interface
- 1.3.1 The Interactive Cycle
1.3.2 The Interactive Porthole
1.3.3 The Interface Design Process
1.4 Summary
Chapter 2 Designing the Functional Model
2.1 Examples of Task-Oriented Functional Design
- 2.1.1 Line Oriented vs. Full-Screen Text Editors
2.1.2 Word Processors
2.1.3 Why Do Secretaries Have Typewriters?
2.2 Overall Approach
- 2.2.1 Task Analysis
2.2.2 Evaluation of the Analysis
2.2.3 Functional Design
2.3 Task Analysis
- 2.3.1 Examples of Task Analysis
2.3.2 VCR Task Analysis
2.3.3 Student Registration Task Analysis
2.4 Evaluation of the Analysis
- 2.4.1 Understanding the User
2.4.2 Goals
2.4.3 Scenarios
2.4.4 Programmers and User Interface Design
2.5 Functional Design
- 2.5.1 Assignment of Agency
2.5.2 Object-Oriented Functional Design
2.6 Summary
Chapter 3 Basic Computer Graphics
3.1 Models for Images
- 3.1.1 Stroke Model
3.1.2 Pixel Model
3.1.3 Region Model
3.2 Coordinate Systems
- 3.2.1 Device Coordinates
3.2.2 Physical Coordinates
3.2.3 Model Coordinates
3.2.4 Interactive Coordinates
3.3 Human Visual Properties
- 3.3.1 Update Rates
3.4 Graphics Hardware
- 3.4.1 Frame Buffer Architecture
3.4.2 Cathode Ray Tube
3.4.3 Liquid Crystal Display
3.4.4 Hardcopy Devices
3.5 Abstract Canvas Class
- 3.5.1 Methods and Properties
3.6 Drawing
- 3.6.1 Paths
3.6.2 Closed Shapes
3.7 Text
- 3.7.1 Font Selection
3.7.2 Font Information
3.7.3 Drawing Text
3.7.4 Outline vs. Bitmapped Fonts
3.7.5 Character Selection
3.7.6 Complex Strings
3.8 Clipping
- 3.8.1 Regions
3.9 Color
- 3.9.1 Models for Representing Color
3.9.2 Human Color Sensitivity
3.10 Summary
Chapter 4 Basics of Event Handling
4.1 Windowing System
- 4.1.1 Software View of the Windowing System
4.1.2 Window Management
4.1.3 Variations on the Windowing System Model
4.1.4 Windowing Summary
4.2 Window Events
- 4.2.1 Input Events
4.2.2 Windowing Events
4.2.3 Redrawing
4.3 The Main Event Loop
- 4.3.1 Event Queues
4.3.2 Filtering Input Events
4.3.3 How to Quit
4.3.4 Object-Oriented Models of the Event Loop
4.4 Event Dispatching and Handling
- 4.4.1 Dispatching Events
4.4.2 Simple Event Handling
4.4.3 Object-Oriented Event Handling
4.5 Communication between Objects
- 4.5.1 Simple Callback Model
4.5.2 Parent Notification Model
4.5.3 Object Connections Model
4.6 Summary
Chapter 5 Basic Interaction
5.1 Introduction to Basic Interaction
- 5.1.1 Functional Model
5.2 Model-View-Controller Architecture
- 5.2.1 The Problem with Multiple Parts
5.2.2 Changing the Display
5.2.3 General Event Flow
5.3 Model Implementation
- 5.3.1 Circuit Class
5.3.2 CircuitView Class
5.3.3 View Notification in the Circuit Class
5.3.4 Overview of the Circuit Class
5.4 View/Controller Implementation
- 5.4.1 PartListView Class
5.4.2 LayoutView Class
5.5 Review of Important Concepts
- 5.5.1 Functional Model
5.5.2 View Notification
5.5.3 View Implementation
5.6 An Alternative Implementation
5.7 Visual C++
- 5.7.1 CView
5.7.2 CDocument
5.8 Summary
Chapter 6 Widget Tool Kits
6.1 Model-View-Controller
- 6.1.1 Widget Models
6.1.2 Independence of View and Controller
6.2 Abstract Devices
- 6.2.1 Acquire and Release
6.2.2 Enable and Disable
6.2.3 Active and Inactive
6.2.4 Echo
6.3 Look and Feel
6.4 The Look
- 6.4.1 What the Look Must Present
6.4.2 Economy of Screen Space
6.4.3 Consistent Look
6.4.4 Architectural Issues in Designing the Look
6.5 The Feel
- 6.5.1 The Alphabet of Interactive Behaviors
6.5.2 Perceived Safety
6.6 Summary
Chapter 7 Interfaces from Widgets
7.1 Data-Driven Widget Implementations
- 7.1.1 Collections of Widgets
7.2 Specifying Resources
- 7.2.1 Resource Organizations
7.2.2 Interface Design Tools
7.3 Layout
- 7.3.1 Fixed-Position Layout
7.3.2 Struts and Springs
7.3.3 Intrinsic Size
7.3.4 Variable Intrinsic Size
7.4 Communication
- 7.4.1 Parent Notification
7.5 Summary
Chapter 8 Input Syntax
8.1 Syntax Description Languages
- 8.1.1 Fields and Conditions
8.1.2 Special Types of Fields and Conditions
8.1.3 Productions
8.1.4 Input Sequences
8.2 Buttons
- 8.2.1 Check Buttons
8.3 Scroll Bars
8. 4 Menus
8.5 Text Box
8.6 From Specification to Implementation
- 8.6.1 Fields
8.6.2 Productions
8.7 Summary
Chapter 9 Geometry of Shapes
9.1 The Geometry of Interacting with Shapes
- 9.1.1 Scan Conversion
9.1.2 Distance from a Point to an Object
9.1.3 Bounds of an Object
9.1.4 Nearest Point on an Object
9.1.5 Intersections
9.1.6 Inside/Outside
9.2 Geometric Equations
- 9.2.1 Implicit Equations
9.2.2 Parametric Equations
9.3 Path-Defined Shapes
- 9.3.1 Lines
9.3.2 Circles
9.3.3 Arcs
9.3.4 Ellipses and Elliptical Arcs
9.3.5 Curves
9.3.6 Piecewise Path Objects
9.4 Filled Shapes
- 9.4.1 Rectangles
9.4.2 Circles and Ellipses
9.4.3 Pie Shapes
9.4.4 Boundary-Defined Shapes
9.5 Summary
Chapter 10 Geometric Transformations
10.1 The Three Basic Transformations
- 10.1.1 Translation
10.1.2 Scaling
10.1.3 Rotation
10.1.4 Combinations
10.2 Homogeneous Coordinates
- 10.2.1 Introduction to the Homogeneous Coordinates Model
10.2.2 Concatenation
10.2.3 Vectors
10.2.4 Inverse Transformations
10.2.5 Transformation about an Arbitrary Point
10.2.6 Generalized Three-Point Transformation
10.3 A Viewing Transformation
- 10.3.1 Effects of Windowing
10.3.2 Alternative Controls of Viewing
10.4 Hierarchical Models
- 10.4.1 Standard Scale, Rotate, Translate Sequence
10.5 Transformations and the Canvas
- 10.5.1 Manipulating the Current Transformation
10.5.2 Modeling with Display Procedures
10.6 Summary
Chapter 11 Interacting with Geometry
11.1 Input Coordinates
11.2 Object Control Points
11.3 Creating Objects
- 11.3.1 Line Paths
11.3.2 Splines
11.3.3 Polygons
11.4 Manipulating Objects
- 11.4.1 Selection and Dragging
11.4.2 General Control Point Dragging Dialog
11.5 Transforming Objects
- 11.5.1 Transformable Representations of Shapes
11.5.2 Interactive Specification of the Basic Transformations
11.5.3 Snapping
11.6 Grouping Objects
- 11.6.1 Selection in a Hierarchical Model
11.6.2 Level of Interaction in a Hierarchy
11.7 Summary
Chapter 12 Drawing Architectures
12.1 Basic Drawing Interface
12.2 Interface Architecture
- 12.2.1 Draw-Area Architecture
12.2.2 Palette Architecture
12.2.3 Summary of Architecture
12.3 Tasks
- 12.3.1 Redrawing
12.3.2 Creating a New Object
12.3.3 Selecting Objects
12.3.4 Dragging Objects
12.3.5 Setting Attributes
12.3.6 Manipulating Control Points
12.4 Summary
Chapter 13 Cut, Copy, and Paste
13.1 Clipboards
- 13.1.1 Simple Clipboard
13.2 Publish and Subscribe
13.3 Embedded Editing
- 13.3.1 Embedded Pasting
13.3.2 Edit Aside
13.3.3 Edit in Place
13.4 Summary
Chapter 14 Monitoring the Interface: Undo, Groupware, and Macros
14.1 Undo/Redo
- 14.1.1 Simple History Architecture
14.1.2 Selective Undo
14.1.3 Hierarchical Undo
14.1.4 Review of Undo Architectural Needs
14.2 Groupware
- 14.2.1 Asynchronous Group Work
14.2.2 Synchronous Group Work
14.2.3 Groupware Architectural Issues
14.3 Macros
14.4 Monitoring Architecture
- 14.4.1 Command Objects
14.4.2 Extended Command Objects
14.5 Summary
Endnotes
Index
- Edition: 1
- Published: July 1, 1998
- Imprint: Morgan Kaufmann
- Language: English
DO
Dan R. Olsen
University Pennsylvania in 1981. He is also the author of User Interface Management Systems. Dr. Olsen has considerable expertise in user interface mangement systems (UIMS), computer graphics, and the construction of compiled and interpreted languages